I haven’t been blogging for a while, but recently, I ran into this challenge while working with a banking customer and thought it would be worth sharing with the community.
This deep dive explores how to implement a Single Pane of Glass (SPOG) solution in Dynamics 365 Customer Service using the Microsoft Authentication Library (MSAL).
I break down how to securely manage tokens, optimize authentication for embedded applications, and tackle common roadblocks—like iframe authentication issues and third-party cookie restrictions. To make it even more practical, I’ve included real-world case studies from finance, banking, and insurance, showcasing successful SPOG deployments, lessons learned, and proven solutions.
You’ll also find detailed architecture diagrams, code samples, and insights into emerging trends, such as AI-driven authentication and next-gen cloud security.
Introduction
SPOG refers to a unified interface where users can access multiple tools and data sources through a single application window. In a Dynamics 365 Customer Service context, a SPOG approach might involve embedding external web applications (knowledge bases, core banking systems, etc.) directly into the Dynamics interface so that service agents have all information at their fingertips. Achieving this unified experience securely requires robust authentication and authorization, ensuring that the embedded applications and Dynamics 365 share a common identity context. Microsoft Authentication Library (MSAL) is Microsoft’s recommended library for handling authentication to Microsoft Entra ID (formerly Azure AD), enabling OAuth 2.0/OIDC flows to acquire tokens for secure API calls. In this post, we refine and expand on SPOG implementation considerations, focusing on OAuth 2.0 with PKCE, token security, MSAL optimizations, and integration patterns.
Deep Dive: OAuth 2.0 with PKCE and MSAL
OAuth 2.0 Authorization Code Flow: Modern authentication in Microsoft Entra ID typically uses the OAuth 2.0 authorization code grant, where the client app redirects the user to a login page and receives an authorization code that it redeems for tokens. In our scenario, the client application could be a custom web app embedded in Dynamics 365. The authorization code flow issues an ID token (for user identity) and an access token (for API access) upon successful login, and optionally a refresh token for obtaining new access tokens without user interaction. The ID token is a JWT containing user claims (name, email, etc.), and the access token grants access to protected APIs (such as Dynamics 365 Web API or external APIs). Using MSAL abstracts much of this protocol – MSAL will handle redirecting to the Microsoft identity platform, caching tokens, and refreshing them as needed.
Proof Key for Code Exchange (PKCE): Single-page applications and mobile apps cannot safely store a client secret, so OAuth 2.0 mandates using PKCE for extra security. PKCE (defined in RFC 7636) is an extension to the auth code flow that mitigates authorization code interception attacks. The client generates a random secret code_verifier and derives a code_challenge (usually a SHA-256 hash) which it sends in the initial authorization request. After the user authenticates, the authorization server (Microsoft Entra ID) issues an authorization code tied to that challenge. The client must present the original code_verifier when redeeming the code for tokens; the server hashes it and compares to the stored challenge to confirm it’s the same client. This means if an attacker somehow intercepts the authorization code, it’s useless without the code_verifier. PKCE thus prevents malicious apps from stealing codes and obtaining tokens. MSAL JS v2+ automatically uses PKCE for SPAs, so developers get this security by default.
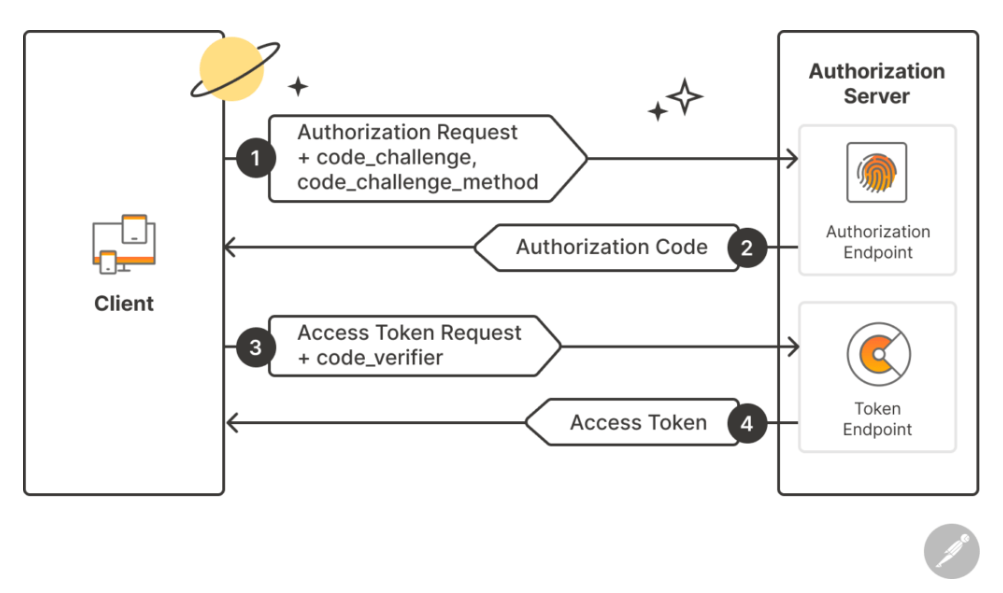
Figure: OAuth 2.0 Authorization Code flow with PKCE (public client with no client secret). The client app initiates an authorization request (1) with a PKCE code challenge. After user login, an authorization code is returned (2). The client redeems the code with the code_verifier (3) to receive tokens (4). Only the app that possesses the code_verifier can exchange the code for tokens
OpenID Connect and ID Tokens: In addition to OAuth 2.0, SPOG scenarios often leverage OpenID Connect (OIDC) for user authentication. OIDC builds on OAuth 2.0 to provide an ID Token — a JWT that MSAL can decode to get user identity information (like name, username, tenant ID, etc.). This is useful for personalization within the embedded apps (e.g. showing the agent’s name or role). MSAL will request scopes like openid profile email
to get ID tokens alongside access tokens. It’s important not to confuse ID tokens (for client app’s user session) with access tokens (for API authorization) – both must be handled correctly but should not be used interchangeably. A best practice is to use the ID token for client-side identity info and include the access token only in calls to the intended APIs.
Token Storage Best Practices and Security Considerations
Token Caching and Lifetime: A core principle for performance and resilience is to cache tokens so that you don’t fetch new tokens unnecessarily. MSAL maintains an in-memory or persistent cache of tokens (ID, access, refresh) keyed by user account. When an access token is needed, MSAL’s acquireTokenSilent()
will return a cached token if it’s still valid, avoiding an HTTP request. Access tokens from Entra ID typically last 1 hour (with adjustable lifetimes), and MSAL will transparently use a refresh token or silent re-authentication to get a new token when needed. By caching tokens and using refresh tokens, users can remain logged in without frequent prompts, and network calls are reduced. In a SPOG scenario, this means an embedded app can quickly retrieve a token to call an external service (e.g., a banking API) without redirecting the user each time, as long as a valid token is in cache.
Secure Token Storage: With great power (tokens) comes great responsibility. Tokens grant access to sensitive data, so how and where they are stored is critical. For web applications, never store tokens in plaintext or in insecure contexts. MSAL.js offers options for cache location: sessionStorage
(default for MSAL v2) or localStorage
, or in-memory. Using sessionStorage is often recommended for SPAs to mitigate the risk of other tabs or scripts accessing the token, though it means tokens won’t persist after the tab is closed. LocalStorage persists across sessions but is accessible by any script on the page (vulnerable to XSS). If using localStorage for SSO across tabs, ensure your app is thoroughly hardened against XSS. Do not store refresh tokens or sensitive tokens in browser localStorage unless absolutely necessary – consider storing them in http-only cookies or relying on MSAL’s built-in mechanisms. As a rule, encrypt token caches at rest and restrict access. For example, in a server-side app, you might encrypt the serialized MSAL token cache and store it in a database or Azure Key Vault. Even in client-side, MSAL will isolate caches per app domain and user, but you should design the app such that each user session uses a separate cache (to avoid token mix-up between accounts). Limit the lifetime of persistent tokens and honor the expires_in
values to proactively refresh or log out users when needed.
Token Replay and Storage Hardening: Implement anti-replay protections for tokens. ID and access tokens are JWTs that the app can validate, but if an attacker steals a token, they could replay it until expiration. MSAL and Azure AD support proof of possession tokens and Continuous Access Evaluation (CAE) – emerging features that can mitigate replay and revoke tokens in real-time, respectively. While these are advanced topics, architects should be aware of them as future enhancements to token security. At minimum, always use HTTPS for any token transport, set strong Content Security Policy (CSP) to prevent injection attacks, and employ secure frameworks to reduce XSS opportunities. Avoid writing tokens to logs or exposing them in URLs. Finally, prefer short-lived access tokens and refresh tokens with adaptive lifetimes (Entra ID gives 24-hour refresh tokens for SPA by default) so that even if stolen, their window of abuse is limited.
Consent and Least Privilege: As part of OAuth, users (or admins via admin consent) must consent to the scopes your embedded app requests. Only request the minimal scopes needed (e.g., if the embedded widget just needs to call one API, don’t ask for broad Graph permissions). Use dynamic scope techniques if possible to adjust permissions on the fly. This reduces security risk and builds user trust. Regularly review and prune unused app permissions in Entra ID.
Performance Optimizations for MSAL and Embedded Apps
Caching and Silent Auth: As noted, caching tokens is the primary performance boost – it avoids needless round-trips. Ensure that MSAL’s cache is used effectively: call acquireTokenSilent()
first whenever you need a token. Only fall back to an interactive method if the silent call fails with an interaction_required
error (MSAL raises an exception like MsalUiRequiredException
in .NET or returns an error in JS). A recommended pattern is “silent, then interactive” – try silent acquisition, and if needed, use a popup or redirect for interactive login. MSAL implements this pattern internally for you to an extent (for example, acquireTokenSilent
will automatically use a stored refresh token if available). By minimizing interactive logins, the user experience is smoother and the load on the identity provider is reduced.
Token Lifetime and Refresh Strategies: Performance can degrade if your app constantly refreshes tokens. Each token issuance is a network call and may involve cryptographic operations. Use the token’s expires_in
and renew only when necessary. MSAL’s cache entries include expiration times and it will proactively use the refresh token some minutes before expiration (typically MSAL tries to refresh in the background or on demand). Avoid explicitly calling acquireTokenSilent
in tight loops or on every API call; instead, retrieve a token once and reuse it for a batch of operations until it nears expiry. Also be mindful of throttling: if an app misuses the token endpoint, Azure AD can return HTTP 429 errors (Too Many Requests). If you ever hit a 429 or 5xx, implement exponential backoff and ensure your caching is working properly. Frequent 429s often indicate the app isn’t caching tokens as it should.
Optimizing MSAL in JavaScript: Newer versions of MSAL (MSAL 2.x for browser) have made significant performance improvements by using the auth code flow + PKCE and having a smaller bundle size than earlier implicit-flow libraries. Still, load time can matter. Only import the MSAL modules you need. For example, if you’re using MSAL in a modular build, import just the PublicClientApplication
from @azure/msal-browser
instead of the whole library. Use the telemetry and performance APIs in MSAL to measure authentication times if needed. MSAL provides hooks (addPerformanceCallback
) to track how long silent token acquisitions or interactive logins take, which can help identify bottlenecks.
Iframe and Popup Performance: In a SPOG scenario, it’s common to embed a web application via an iframe in the Dynamics 365 form or dashboard. This introduces unique performance considerations. Traditionally, some apps would attempt silent SSO by loading an auth iframe with prompt=none
to reuse an existing login session. However, with modern browsers blocking third-party cookies, this approach often fails. The alternative, as recommended by Microsoft, is to use the authorization code + refresh token flow (via MSAL 2.x) to avoid relying on hidden iframes for silent auth. When an embedded app loads, if the user is already logged into Entra ID (i.e., has a valid session cookie), MSAL can directly perform a full-page redirect and back to get tokens without user credentials (the user won’t see a login prompt). This may reload the SPA, but can be done quickly if the app is cached in the browser. To optimize, consider initiating this redirect early in the app load (perhaps on a splash screen or initial load sequence) so that by the time the user interacts, the authentication is done. Alternatively, MSAL’s loginPopup()
can be used so the parent Dynamics page is not reloaded – a small popup window handles the redirect and token acquisition. Popups can be tricky (browsers might block them unless triggered by user interaction), so ensure you open the popup in response to a user gesture or use a timeout. The redirect vs popup trade-off is important: redirects are more foolproof and work even if popups are blocked, but they reload the app; popups preserve SPA state, but can be blocked and add complexity. Evaluate which yields better UX in your scenario.
Resource Loading in Embedded Apps: Another performance tip for SPOG: if you’re embedding multiple iframes or web resources in a Dynamics form, load them on demand. Dynamics 365 allows form tabs to be collapsed or lazy-loaded – for example, an iframe web resource can be set to load only when the user navigates to that tab. This prevents slowing down the initial form load with numerous embedded apps (some of which the agent might not even use every time). For instance, if an agent only sometimes opens a “Maps” widget or a “Credit Check” panel, consider loading those on click rather than all at once. There are documented techniques to load iframes asynchronously to improve CRM form performance. Coupling this with MSAL SSO means that the first time an iframe app is loaded, it might do a quick silent auth (which if configured correctly, should not prompt the user) and then be ready.
Network and CPU Considerations: MSAL uses cryptography for PKCE (SHA-256 hashing the code verifier) and for token signature verification in some cases. These are usually negligible on modern devices, but on lower-end hardware (or mobile browsers) there is a minor CPU cost. It’s generally not an issue, but be aware if your embedded app runs on, say, a kiosk with limited resources. Also ensure gzip compression is enabled for all JS libraries (including MSAL) to reduce transfer time.
Embedding Applications in Dynamics 365 – Architecture and Security
When integrating an external application into Dynamics 365 Customer Service (for example, via an iframe on a dashboard or form), the architecture involves multiple layers that must work in harmony: the Dynamics 365 host page, the embedded app, and the identity provider (Entra ID). The diagram below outlines a typical flow for an embedded SPOG component using MSAL:
- User Login to Dynamics 365: The user signs into Dynamics 365 Customer Service via Entra ID (OAuth 2.0). This establishes an authenticated session with Entra ID (setting a session cookie in the browser for
login.microsoftonline.com
orlogin.microsoft.com
) and with Dynamics 365. At this point, the user has an Entra ID session and a valid ID/access token that Dynamics itself uses under the hood. Dynamics 365 might use its own client-side logic to cache tokens for Dataverse calls, but that’s abstracted from the end user. - Launching Embedded App (Web Resource/IFrame): The user navigates to a part of Dynamics (say a dashboard or a customer form) where an external app is embedded. Dynamics 365 loads the iframe content (which could be a web resource hosted within Dynamics, or an external URL if allowed). For security, the embedded content is typically constrained by same-origin policies. If it’s an external URL, ensure the Content Security Policy of Dynamics and the X-Frame-Options of the external site are configured to permit this embed (more on that below).
- MSAL Initialization in Embedded App: The embedded application (running in the iframe) initializes MSAL to prepare for authentication. It uses its own Azure AD App Registration (with a client ID distinct from Dynamics 365’s ID) configured for SPA redirect URIs. In MSAL configuration, it specifies the
clientId
,authority
(tenant or common), and aredirectUri
which is typically a blank page or a page within the app that MSAL can redirect to during the auth code flow. For example:
const msalConfig = {
auth: {
clientId: "",
authority: "https://login.microsoftonline.com/", // or 'common' for multi-tenant
redirectUri: "/authComplete.html" // a page that calls MSAL.handleRedirectPromise()
},
cache: {
cacheLocation: "localStorage", // to share SSO state across sessions/tabs if needed
storeAuthStateInCookie: false // set true if dealing with older browsers or iframe issues
}
};
const msalInstance = new msal.PublicClientApplication(msalConfig);
In the above snippet, we choose localStorage for cache to allow persistence and potential SSO across tabs (with the trade-off of needing strong XSS protections). The redirectUri
is an important page – it should be a minimal page that just invokes MSAL to handle the redirect response (processing the auth code and obtaining tokens). In Dynamics CRM web resources, this might need to be a page deployed as part of the solution. Ensure this redirect page is accessible (e.g., if the app is on a different domain, that domain’s redirect needs to be in Azure AD app registration and allowed by CSP).
Single Sign-On via SSO Silent or Popup: Now the embedded app needs to authenticate the user. Since the user is very likely already logged in to Entra ID (from step 1), we want to do this without prompting the user again. MSAL offers two primary ways to achieve SSO in this context: ssoSilent()
and loginPopup()
(with an account hint).
- Silent SSO (preferred): MSAL’s
ssoSilent()
can attempt to sign-in a user based on an existing session cookie. The embedded app can request an ID or access token silently. Under the hood, MSAL will open an iframe to the /authorize endpoint withprompt=none
and try to acquire an auth code using the existing AAD session cookie. If the user has a single account session, this can succeed and yield tokens silently. To increase the success rate, we supply a hint about the user: either their login username (loginHint
), their Entra ID account object (account
object from a parent MSAL cache), or a Session ID (sid) if known. In a SPOG setup, we often know who the user is from the parent context (Dynamics). For example, Dynamics 365 can surface the user’s login name or object ID via its web APIs. We can pass this as a query parameter to the iframe URL or viapostMessage
. The iframe app then uses it in MSAL’s silent request:
// Assuming loginHint was passed in URL or via window.name, etc.
msalInstance.ssoSilent({
loginHint: userEmail,
scopes: ["api:///.default"] // or other scopes needed
}).then(response => {
// SSO succeeded, we have response.account and response.accessToken
}).catch(err => {
if (err instanceof msal.InteractionRequiredAuthError) {
// Silent SSO failed (perhaps cookie blocked or multiple sessions), fallback
msalInstance.loginPopup({…});
} else {
console.error(err);
}
});
In the code above, we attempt silent SSO with a loginHint. If the user’s session is fresh and single, no UI is shown and we get a token. If it fails (e.g., in Safari where third-party cookies are blocked, or if the user has multiple accounts and AAD doesn’t know which to use), we catch an InteractionRequired
error. In that case, we then use loginPopup()
as a graceful fallback.
Popup with Account Selection: By calling msalInstance.loginPopup({ scopes, loginHint })
, MSAL will open the AAD login in a centered popup. If the user has an active session, AAD might directly redirect back with tokens, or it might show an account picker if multiple accounts are in session. This requires the Dynamics host page to allow popups from the iframe. If the iframe is sandboxed (in some contexts Dynamics might sandbox iframe content), you must include the allow-popups
flag in the sandbox attribute. The MSAL documentation notes that interactive auth is blocked in iframes by default (X-Frame-Options DENY on the AAD login page), hence the need for a popup which is a top-level window. The popup will perform the redirect to the Entra ID login (carrying the PKCE code challenge, etc.), then eventually redirect to the provided redirectUri (e.g., authComplete.html
) within that popup. MSAL listens for the result and closes the popup, returning the AuthenticationResult
to the calling code. This approach avoids a full-page redirect of the Dynamics window and can be a good user experience if done quickly after an initial attempt. The downside is a popup window momentarily appearing—on a fast network this might be just a flash.
Token Acquisition and Usage: Once the embedded app obtains the access token, it can call whatever API or service it’s designed to integrate. For instance, a banking SPOG component might call an external REST API to fetch customer financial data, or a knowledge base widget might call a search service. The access token should be scoped for that backend (e.g., issued for a custom API or Microsoft Graph, etc., depending on the use case). Using MSAL ensures the token is stored in its cache. The embedded app should handle token expiration by again using acquireTokenSilent
(which will use the refresh token if available, or attempt another silent auth) before the token expires, so the user remains seamlessly connected.
Logout and Session Management: In an integrated SPOG environment, logout needs special care. If a user signs out of Dynamics 365, ideally the embedded apps should also sign out (and vice versa). One approach is to have the embedded app listen for the parent window’s logout event (if any) or the parent could invoke a script on iframes to clear their session. MSAL provides logoutPopup()
which can be called in an embedded context to sign the user out without full redirect. However, a full single-sign-out across multiple apps in the browser is non-trivial – the user may remain logged into Entra ID in their browser, so logging out from one app doesn’t automatically log them out from others unless you specifically invoke a logout for each. For high-security scenarios, consider implementing front-channel or back-channel logout notifications between the SPOG container and the apps.
Secure IFrame Integration: Embedding applications raises clickjacking and content security concerns. By default, Azure AD’s login page will not render in an iframe (to prevent clickjacking attacks). This is why MSAL disallows redirect
inside iframes unless you explicitly enable the (not recommended) allowRedirectInIframe
setting for special cases like Azure AD B2C custom pages. For line-of-business apps, stick to the popup method for interactive auth to avoid this. Additionally, if your embedded app is on a different domain than Dynamics (which it likely is, since Dynamics is on <tenant>.dynamics.com and your app might be on your corporate domain or a cloud service domain), you must configure the embedded app’s HTTP response headers to allow being framed by the Dynamics domain. Use the X-Frame-Options: ALLOW-FROM https://<yourDynamics>.dynamics.com
header or, more modernly, a Content-Security-Policy directive: Content-Security-Policy: frame-ancestors https://<yourDynamics>.dynamics.com
. If you control the embedded app’s code, implement this header to prevent other sites from framing it while explicitly permitting your Dynamics 365 instance. Note that Dynamics 365 itself has started adopting CSP headers – by default model-driven apps send a CSP that includes frame-ancestors 'self' https://*.powerapps.com
. If your embedded app is hosted under a different domain (not powerapps.com and not the same origin as Dynamics), you may need to adjust the Power Platform admin settings to allow additional frame sources or disable CSP for that org (the CSP can be customized or turned off in the Power Platform Admin Center). Be cautious and test thoroughly if adjusting CSP to ensure you’re not inadvertently exposing the app to clickjacking from unapproved domains.
Cross-Domain SSO Challenges: As mentioned, modern browsers treat the AAD session cookie as a third-party cookie when an app is in an iframe. Many browsers (Safari, Firefox, and Chrome in incognito or with certain settings) block third-party cookies by default. This means even if the user is logged into AAD, the iframe might not see that cookie, causing ssoSilent
to fail. MSAL anticipates this: if it detects no access to local storage or cookies in the iframe, it falls back to in-memory cache. From a user perspective, the likely outcome is they’ll get a popup for login despite having a session. To minimize disruption, design your UI to possibly inform the user or corporate policy to allow certain cookies. For an internal enterprise scenario, you might distribute group policy or documentation for users on how to enable third-party cookies for the Dynamics domain (or use Edge’s Enterprise Site Mode lists, etc., to allow your specific scenario). The Microsoft documentation also suggests a temporary workaround using a redirect or popup on first load – essentially treat the initial authentication as a first-party navigation (so the browser sends the cookie). For example, the embedded app could on load do window.top.location = "https://login.microsoftonline.com/...redirectUri=postMsgBlank.html"
which after auth sets some flag and returns to the Dynamics page or communicates via postMessage. This is complex, so often using loginPopup
triggered by a user gesture is simpler when third-party cookies are blocked.
Code Sample – Passing Session ID from Dynamics to IFrame: One clever technique for SSO in iframes is for the parent (Dynamics) to pass the session ID (sid) of the user’s AAD session to the child. If Dynamics can obtain the user’s sid claim (for example, from its own ID token – though that might require customizing how you get that info, since Dynamics front-end doesn’t expose it by default), the sid can be provided to MSAL. The MSAL iframe documentation shows using ssoSilent({ sid: <sid> })
to let Azure AD know exactly which session to use. The sid is a GUID that identifies the user’s session at AAD. In practice, passing login_hint (user principal name) is often sufficient and easier to get. But in scenarios with multiple sessions or guest users, sid could provide a more direct hint. Below is a code snippet illustrating parent-to-iframe communication using URL query string (one could also use window.postMessage
):
Dynamics (parent page) snippet: (assuming we have the user UPN from context)
<iframe id="spogApp" src="https://embed.contoso.com?loginHint=<%= user.email %>"></iframe>
// On load, read loginHint from URL
const params = new URLSearchParams(window.location.search);
const loginHint = params.get("loginHint");
msalInstance.ssoSilent({ loginHint: loginHint, scopes: ["..."] })
.then(result => { console.log("SSO successful, user:", result.account.username); })
.catch(err => {
console.warn("Silent SSO failed, opening popup");
return msalInstance.loginPopup({ loginHint: loginHint, scopes: ["..."] });
});
This way, if the user’s email matches an active session, Azure will log them in silently. If not, the popup will pre-fill that login hint at the prompt. The overall user experience can be nearly frictionless.
Real-World Case Studies and Lessons Learned
Case Study 1 – Banking Sector (360° Customer View): A multinational bank implemented Dynamics 365 Customer Service to centralize customer inquiries. Agents previously had to switch between the CRM and a core banking portal to verify account balances, loan statuses, etc., leading to slow response times. Using a SPOG approach, the bank embedded a custom web app into Dynamics that displays a “Customer 360” view aggregating data from the core banking system and credit databases. This was achieved by leveraging Azure AD for authentication across both systems. The embedded app uses MSAL to sign in the user with the same Entra ID account that Dynamics uses, obtaining an access token for the banking API. With proper consent and permissions, the token allows read-only access to core banking data. By caching tokens and using silent SSO, the integration feels seamless – when an agent opens a customer record in Dynamics, the embedded panel instantly shows live banking data without an extra login. This SPOG solution yielded significant efficiency gains. In fact, Microsoft’s Banking Accelerator notes that relationship managers benefit from a unified 360° customer form pulling data from multiple sources like CRM and core banking, enabled by integration on the Business Application Platform. The bank saw similar benefits: reduced call handling times and improved user satisfaction as agents had all info on one screen. Challenges: A challenge encountered was handling multi-tenant scenarios – some bank staff had to access data from a partner system in a different AAD tenant. To solve this, the embedded app was made multi-tenant in AAD and used OAuth 2.0 On-Behalf-Of flow for calling downstream APIs across tenants, with the user’s consent. This is an advanced pattern, but it underscores the flexibility of MSAL and Azure AD to enable cross-organization SSO in a SPOG context. The bank’s security team also mandated strict CSP headers. The embedded app’s domain was whitelisted in Dynamics’s frame-ancestors CSP, and conversely the app only allowed the bank’s CRM URL to frame it, preventing any other site from embedding it (mitigating clickjacking).
Case Study 2 – Insurance & Healthcare (Unified Agent Desktop): An insurance company in the UK deployed a unified agent desktop using Dynamics 365 Unified Service Desk (USD) and later the web-based Customer Service Workspace. They needed to integrate policy management, claims processing, and a telephony system into one interface. Using USD (a desktop framework), they could host multiple applications and achieved a “single pane of glass” experience with features like screen pop and click-to-dial. In the move to Dynamics 365 online, they wanted a purely web-based SPOG. They built web counterparts for the policy and claims systems and embedded them in the Dynamics Customer Service Workspace app via iframes. MSAL with OAuth 2.0 was used for authentication to these web systems, using the Azure AD accounts of agents. A notable challenge was ensuring performance and stability – if one embedded system crashed or took too long to load, it could affect the agent’s workflow. They tackled this by implementing a circuit breaker: if an iframe app failed to load or auth within a few seconds, it would show a fallback message and not block the agent’s work on Dynamics (the agent could retry loading that panel if needed). This kind of resilience design is important in SPOG so that one component’s hiccup doesn’t bring down the whole pane. On the security side, the insurance company dealt with strict privacy regulations. All access to customer health data via the embedded app was audited. They used MSAL’s logging in confidential client mode (for server-to-server calls) to trace which tokens were used to call what APIs, mapping that to user activity in the CRM. This provided an audit trail satisfying compliance. The result of this unified desktop was a 25% increase in support efficiency and a 35% reduction in call transfers, as agents could handle most tasks without handing off calls or logging into separate systems.
Case Study 3 – Global IT Support (Microsoft’s Internal Use): Microsoft’s own support division, which serves millions of users, consolidated from 16 different case management tools into Dynamics 365 Customer Service. The goal was a single pane of glass for support engineers. They integrated tools like Teams (for collaboration), internal diagnostics, and customer history into the Dynamics interface. While specifics of their authentication aren’t public, we know they achieved a seamless experience: “Dynamics 365 Customer Service places this information all on a single pane of glass. Engineers don’t have to switch between multiple tools…”. Likely, Azure AD and MSAL played a key role – as engineers log into Dynamics, tokens or SSO were used to bring in data from other internal systems (possibly via Graph API or embedded Power BI dashboards). One can infer challenges they tackled: role-based access – ensuring engineers only see data they’re permitted to. Using Entra ID’s token scopes and Dynamics’ security roles in tandem, they would enforce that an engineer’s token (which might include group/role claims) only allows access to certain information in an embedded frame. For instance, a less privileged support agent might not see the full diagnostic tools pane. They probably used conditional access policies to further secure the overall solution (e.g., requiring MFA for accessing certain sensitive integrated tools, or ensuring the SPOG is only accessible from trusted networks). This case underscores that beyond technology, user training and change management is crucial: consolidating tools into one interface required training engineers used to disparate systems. The payoff was huge – first-call resolution jumped by 31%, indicating the SPOG approach enabled faster problem-solving.
Common Challenges and Solutions: Across these scenarios, a few recurring themes emerged:
- Authentication across organizational boundaries: When integrating systems from different Azure AD tenants (or Azure AD and other IdPs), plan for multi-tenant app registrations or federation. For example, if your Dynamics tenant needs to show data from a partner’s tenant, you may use Azure AD B2B (guest accounts) or have the embedded app support multiple authorities (common endpoint with home tenant discovery). MSAL can be configured for multi-tenant by using
authority: "https://login.microsoftonline.com/organizations"
or similar, and handling the homeAccountId for each user. - Third-Party Cookies: As discussed, this is a biggie for silent SSO. Some real deployments ended up choosing an easier path: instead of embedding the app directly, using a pop-out approach – e.g., the SPOG shows a preview or limited info and when more interaction is needed, it opens the full app in a new tab where SSO is straightforward (first-party context). While this breaks the “single pane” ideal, it sometimes was necessary for advanced web apps that just couldn’t fit in an iframe due to cookie issues. A solution on the horizon is Continuous Access Evaluation (CAE) and Azure AD’s new mechanisms that might allow silently sharing tokens via parent context, but currently the popup or redirect method is the way.
- User Context Sharing: In SPOG, you often want to pass context (like a customer ID) from Dynamics to the embedded app so it knows what data to display. This is not directly an auth issue, but it intersects with security – never trust the context from the parent blindly if the embedded app also serves other purposes. In one case, a company accidentally exposed data by constructing an iframe URL with query like
...?recordId=XYZ
– a user savvy enough could copy that iframe URL and potentially manipulate the recordId to fetch data they shouldn’t (if the embedded app did not re-check permissions). The solution is to treat any such parameters as hints, but always use the access token to do server-side authorization for the data. In other words, the embedded app should call its API with the token and server-side verify that the token’s user is allowed to access record XYZ. Rely on backend authorization, not just front-end passing of IDs. - Performance tuning: Each embedded app adds load time. A tip found useful is to load lighter-weight versions of apps in the SPOG. For example, instead of loading a full CRM inside another CRM (which would be heavy), one might create a lightweight custom page that only shows the needed info (using APIs behind the scenes). Use lazy loading, as mentioned, and consider time-outs – if an app doesn’t load in, say, 5 seconds, show a “Click to retry” to the agent so they can continue with other tasks.
Future Trends and Innovations
Evolution of SPOG in Enterprises: The concept of a single pane of glass is evolving beyond just embedding iframes. Enterprises are looking at composable UX where components from various applications can be dynamically assembled. Microsoft’s introduction of Dynamics 365 Apps like Customer Service Workspace and Omnichannel already point in this direction – these apps allow opening multiple sessions, side panels, and integrated Microsoft Teams chats, all within one screen. The future SPOG might leverage micro-frontend architecture, where each section of the screen is a component that can be independently deployed/updated, communicating with each other through well-defined channels. This could reduce the coupling and allow more scalable development across teams. We also see SPOG expanding to cover not just customer service, but IT management and security operations (for instance, the concept of XDR in security – a single pane for all security alerts). The principles we discussed (OAuth SSO, embedding, caching) will apply similarly, but perhaps frameworks will emerge to simplify it. Microsoft’s Power Platform is heading that way, enabling canvas apps or Power BI tiles to be inserted into model-driven apps (mash-up of UIs). In a few years, a customer service agent’s screen might include native Dynamics components, Power Apps components, and third-party app widgets all unified – potentially hosted in Teams as the shell (since Teams is becoming a common front-end for work). This blurs the lines between what is “Dynamics” and what is an external app, truly achieving SPOG.
AI-Powered Authentication (Entra ID): Microsoft Entra ID is integrating AI in subtle but impactful ways. One area is Adaptive Authentication – the system can assess user risk in real-time (using machine learning on signals like atypical location, unfamiliar device, impossible travel, etc.) and decide if MFA or additional proof is needed. From a developer standpoint using MSAL, this manifests as Conditional Access policies that might trigger an MFA or device compliance check during the token acquisition. MSAL already handles receiving a Conditional Access challenge (like an MFA requirement or CAE claim) by throwing an exception that you handle by redirecting the user to interaction. Going forward, we may see even more seamless handling – e.g., Continuous Access Evaluation (CAE) which allows Entra ID to notify the client that a token is revoked or needs re-auth (perhaps due to an AI-detected risk) mid-session. MSAL has begun supporting CAE so that long-lived tokens can be revoked and renewed on the fly when risk changes. Another AI aspect is anomaly detection and fraud prevention. Microsoft Defender and Entra ID Identity Protection use AI to flag risky sessions. While not directly in MSAL, these feed into what tokens get issued or blocked. For example, if Identity Protection thinks a session is likely a bot, it might require a CAPTCHA or deny the token issuance. In the future, AI-driven authentication could mean the auth flow might adapt in real-time – possibly altering token scopes or requiring step-up auth if an AI judges the requested resource is sensitive in context. Solution architects should keep an eye on features like Entra Verified ID and decentralized identity as well. Microsoft is exploring AI for verifying credentials (e.g., facial matching for ID verification) – in a customer service scenario, an agent might verify a customer’s identity through an AI service, and that could integrate into the SPOG (though that’s more on the customer side than the agent’s auth). Nonetheless, as AI becomes more embedded, we may even see MSAL offering easier integration with biometrics and passwordless methods (FIDO2 keys, Windows Hello). Passkeys (FIDO) are a big trend – at Ignite 2023, Microsoft announced users will soon be able to login with passkeys stored in Authenticator. That means our SPOG apps should be tested with passwordless sign-ins and ensure MSAL can handle WebAuthn flows. Fortunately, MSAL mostly abstracts it – if the user’s account is passwordless, the AAD login page will just invoke the device’s biometric prompt.
Cloud Security and Access Management Enhancements: On the horizon, cloud access management is becoming more granular and automated. For example, Policy Based Access Control (PBAC) or attribute-based access might augment role-based models. Azure AD is introducing more fine-grained admin roles and perhaps app roles that can be assigned via rules. For SPOG, this could allow even more tailored experiences: imagine tokens that carry not just static roles but dynamic attributes (like “tier=gold support”) that the embedded app uses to decide what to show. We also expect better integration between identity and network security. As companies adopt Zero Trust, the network location is no longer implicitly trusted, but signals from Secure Access Service Edge (SASE) or VPN can feed into identity decisions. A concrete trend is coupling Conditional Access with device posture and compliance (e.g., requiring a compliant device for accessing certain SPOG features). We might see MSAL integrated with device attestation in the future. Also, session management improvements: CAE as mentioned, and maybe OAuth 2.1 features or whatever comes next. The OAuth 2.0 Security Best Current Practice RFC is guiding deprecating the implicit flow (already done) and reinforcing PKCE everywhere (done), and suggests shortening token lifetimes and using sender-constrained tokens. Sender-constrained access tokens (bound to a client cert or a proof of possession key) could make their way into MSAL, which would greatly improve security (stolen tokens would be unusable without the key). Though this isn’t mainstream in SPA scenarios yet, Microsoft might leverage the browser (e.g., client TLS or WebCrypto) to bind tokens to the client. For developers, this would be mostly transparent but might require using MSAL libraries that support that and perhaps managing a key pair.
In summary, the future will bring even tighter integrations – Teams as a platform for SPOG, AI-driven context-aware authentication, and continuous authorization will shape how we implement solutions. Solution architects should design today’s systems with an eye on flexibility for these changes: use standard protocols (OAuth/OIDC) via libraries like MSAL so that improvements automatically benefit your app (when MSAL updates to support new features, you get them by updating the package). And always enforce principles of least privilege and strong identity – those won’t change, even as tech evolves.
Key Takeaways
- Use OAuth 2.0 Authorization Code Flow with PKCE for any embedded or SPA applications. This ensures secure token exchange without needing a stored client secret, preventing code interception attacks. MSAL libraries handle PKCE automatically and should be preferred over custom auth code implementations.
- Securely cache and handle tokens to balance security and usability. Cache tokens to avoid unnecessary logins, but isolate token caches per session/user and encrypt persistent stores. Never expose tokens in logs or URLs, and be cautious with localStorage in the browser (consider it only for low-sensitivity tokens or use sessionStorage/in-memory with proper UX trade-offs).
- Architect for silent SSO but prepare fallbacks. Strive for a seamless experience by leveraging existing user sessions with
acquireTokenSilent
orssoSilent
. However, handle exceptions where silent auth fails (due to cookies or multiple sessions) by falling back to interactive flows (popup or redirect). Always test embedded scenarios on different browsers with varying privacy settings. - Optimize performance by minimizing auth round-trips. Utilize MSAL’s token cache and refresh capabilities so that the SPOG components don’t continuously re-authenticate. Load embedded content on-demand and in parallel where possible. Use hints (login_hint or sid) to expedite SSO and reduce the chance of prompts. Monitor for throttling (AAD will throttle excessive token requests – if hit, implement backoff and fix caching).
- Ensure iframe integration is done securely. Configure X-Frame-Options or CSP
frame-ancestors
on all embedded content to allow only trusted domains (e.g., your Dynamics 365 URL). Within Dynamics, if CSP is enabled, update the allowlist to include your domains. Sanitize any data exchanged between parent and iframe, and do not rely solely on the client side for access control. - Learn from real-world SPOG deployments. Common pitfalls include third-party cookie blocking, which can break silent SSO– address this by using popup-based auth or user education for settings. Another is user context mismatch – always verify that the user identified by the token is authorized for the data shown. Use Azure AD groups or app roles in tokens to enforce granular access in embedded apps, especially in cross-team integrations.
- Stay updated on MSAL and Entra ID developments. Regularly update MSAL libraries to benefit from security patches and new features (Microsoft actively updates MSAL – e.g., to improve performance and support CAE). Watch for announcements on Entra ID (Azure AD) regarding improvements like CAE, passkeys support, or policy changes that might affect your auth flow. Plan to support passwordless and MFA as standard – design your SPOG such that an MFA prompt, if triggered, can be handled (usually via an interactive login popup that MSAL will invoke when required by Conditional Access).